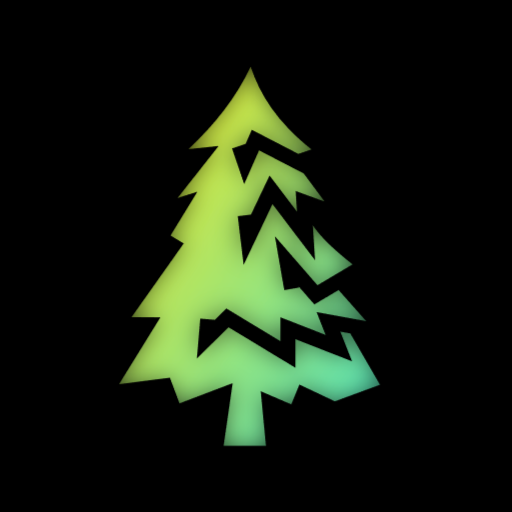
[LANGUAGE: C#]
I kept trying to create clever solutions, but ended up falling back on regex when it was taking to long. THE TLDR is we scan the list of strings for a symbol, then parse the three lines above, below and inline with the symbol for digits. Then we try and match the indexes of the match and the area around the symbol. Part 2 was a small modification, and was mostly about getting the existing code to conform the data into a pattern for each of the three lines.
Part 1
static char[] Symbols = { '@', '#', '$', '%', '&', '*', '/', '+', '-', '=' };
string pattern = @"\d+";
static List? list;
list = new List((await File.ReadAllLinesAsync(@".\Day 3\PuzzleInput.txt")));
int count = 0;
for (int row = 0; row < list.Count; row++)
{
for (int col = 0; col < list[row].Length; col++)
{
var c = list[row][col];
if (c == '.')
{
continue;
}
if (Symbols.Contains(c))
{
var res = Calculate(list[row - 1], col);
res += Calculate(list[row], col);
res += Calculate(list[row + 1], col);
count += res;
}
}
}
Console.WriteLine(count);
private static int Calculate(string line, int col)
{
List indexesToCheck = new List { col - 1, col, col + 1 };
int count = 0;
MatchCollection matches = Regex.Matches(line, pattern);
foreach (Match match in matches)
{
string number = match.Value;
if (AnyIndexInList(indexesToCheck, match.Index, match.Length))
{
count += Int32.Parse(number);
}
}
return count;
}
static bool AnyIndexInList(List list, int startIndex, int length)
{
for (int i = startIndex; i < startIndex + length; i++)
{
if (list.Contains(i))
{
return true;
}
}
return false;
}
Part 2:
list = new List((await File.ReadAllLinesAsync(@".\Day 3\PuzzleInput.txt")));
int count = 0;
for (int row = 0; row < list.Count; row++)
{
for (int col = 0; col < list[row].Length; col++)
{
var c = list[row][col];
if (c == '.')
continue;
if (c == '*')
{
var res1 = Calculate2(list[row - 1], col);
var res2 = Calculate2(list[row], col);
var res3 = Calculate2(list[row + 1], col);
count += (res1, res2, res3) switch
{
{res1: not null, res2: null, res3: null } when res1[1] != null => res1[0].Value * res1[1].Value,
{res1: null, res2: not null, res3: null } when res2[1] != null => res2[0].Value * res2[1].Value,
{res1: null, res2: null, res3: not null } when res3[1] != null => res3[0].Value * res3[1].Value,
{res1: not null, res2: not null, res3: null } => res1[0].Value * res2[0].Value,
{res1: not null, res2: null, res3: not null } => res1[0].Value * res3[0].Value,
{res1: null, res2: not null, res3: not null } => res2[0].Value * res3[0].Value,
{res1: not null, res2: not null, res3: not null } => res1[0].Value * res2[0].Value * res3[0].Value,
_ => 0
} ;
}
}
}
Console.WriteLine(count);
private static int?[]? Calculate2(string line, int col)
{
List indexesToCheck = new List { col - 1, col, col + 1 };
int?[]? count = null;
MatchCollection matches = Regex.Matches(line, pattern);
foreach (Match match in matches)
{
string number = match.Value;
if (AnyIndexInList(indexesToCheck, match.Index, match.Length))
{
if (count == null)
count = new int?[2] { Int32.Parse(number), null };
else {
count[1] = Int32.Parse(number);
};
}
}
return count;
}
To list some good ones that haven't already been ssid:
Stop podcasting yourself
A problem squared
Secretly Incredibly Fascinating